DECLARE
/* @STRQUERY AND @PARAMDEF SHOULD BE NVARCHAR
BECAUSE "EXECUTE sp_executesql" ACCEPTS STATEMENT AND PARAMETER OF TYPE 'ntext/nchar/nvarchar' ONLY
OTHERWISE
"Procedure expects parameter '@statement' of type 'ntext/nchar/nvarchar'."
OR
"Procedure expects parameter '@parameters' of type 'ntext/nchar/nvarchar'." WILL BE RAISED*/
@STRQUERY AS NVARCHAR(200),
@STRNAME AS VARCHAR(100),
@PARAMDEF AS NVARCHAR(100),
@STRNAMEVALUE AS VARCHAR(100)
BEGIN
--FOLLOWING STATEMENT ASSING VALUE
SET @STRNAMEVALUE='MANINDER'
--FOLLOWING VARIABLE CONTAINS A QUERY WHICH USES A PARAMETER
SET @STRQUERY = 'SELECT ''YOUR NAME IS '' + @STRNAME'
--FOLLOWING STATEMENT DEFINES THE PARAMETERS USED IN THE QUERY
SET @PARAMDEF = '@STRNAME AS VARCHAR(100)'
--IN THE FOLLOWING STATEMENT "@STRNAME = @STRNAMEVALUE" STATEMENT ASSIGNS VALUE TO THE PARAMETER
--SYNTAX IS EXECUTE sp_executesql @STATEMENT, @PARAMETERS
EXECUTE sp_executesql @STRQUERY, @PARAMDEF,@STRNAME = @STRNAMEVALUE;
END
Shankey
Saturday, November 20, 2010
A look at the difference between Convert.ToString() and .ToString() method.
Below example clears the confusion between the usage of both the method. Thus developers will it appropriately
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
namespace ExampleOfString
{
class Program
{
static void Main(string[] args)
{
String UserName = null ;
try
{
//Below statement WILL THROW an exception as .ToString() does not handles null values
String strUserId = UserName.ToString();
}
catch (NullReferenceException ex)
{
Console.WriteLine("Error:{0}", ex.Message);
}
try
{
//Below statement WILL NOT THROW an exception as convert.tostring handles implicitly
String strUserId = Convert.ToString(UserName);
Console.WriteLine("UserName:{0}", strUserId);
}
catch (NullReferenceException ex)
{
Console.WriteLine("Error:{0}", ex.Message);
}
}
}
}
/*
Below is the output of the console application
Error:Object reference not set to an instance of an object.
UserName:
Press any key to continue . . .
*/
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
namespace ExampleOfString
{
class Program
{
static void Main(string[] args)
{
String UserName = null ;
try
{
//Below statement WILL THROW an exception as .ToString() does not handles null values
String strUserId = UserName.ToString();
}
catch (NullReferenceException ex)
{
Console.WriteLine("Error:{0}", ex.Message);
}
try
{
//Below statement WILL NOT THROW an exception as convert.tostring handles implicitly
String strUserId = Convert.ToString(UserName);
Console.WriteLine("UserName:{0}", strUserId);
}
catch (NullReferenceException ex)
{
Console.WriteLine("Error:{0}", ex.Message);
}
}
}
}
/*
Below is the output of the console application
Error:Object reference not set to an instance of an object.
UserName:
Press any key to continue . . .
*/
Tuesday, November 2, 2010
Trim function in javascript to replaces leading and trailing spaces
A simple trim function in javascript, which replaces leading and trailing spaces.
You can define a trim function which trims leading and trailing space in javascript by using any one of the following way:
way 1:
way 2:
Following function shows how to use the trim function
you will see 'maninder' in the alert box. All the leading and trailing space are trimmed
You can define a trim function which trims leading and trailing space in javascript by using any one of the following way:
way 1:
String.prototype.trim = function()
{ return this.replace(/^\s+|\s+$/g, ''); }
way 2:
function trim() { return this.replace(/^\s+|\s+$/g, ''); }
Following function shows how to use the trim function
function temp() { var str=' maninder '; alert(str.trim()); }
you will see 'maninder' in the alert box. All the leading and trailing space are trimmed
Saturday, October 30, 2010
How to enable debugging or attach debugger in visual studio with silverlight when browser is firefox
This will help all the people who are facing problem to attaching the debugger of visual studio when firefox is use for browsing.
Today I was facing problem in attaching visual studio debugger while using firefox. I kept googling here and there and then almost spending 3-4 hours, Some where i found that when you use IE then your debugger will get attached. I tried it and the problem was solved. Finally the debugger got attached with IE and not with firefox. Then further googling gave me following solution in which you have change the config of the firefox
Solution:
Thanks,
Maninder
Today I was facing problem in attaching visual studio debugger while using firefox. I kept googling here and there and then almost spending 3-4 hours, Some where i found that when you use IE then your debugger will get attached. I tried it and the problem was solved. Finally the debugger got attached with IE and not with firefox. Then further googling gave me following solution in which you have change the config of the firefox
Solution:
- In address bar of FireFox type "about:config".
- Read the warning and accept it.
- Now type: "npctrl" in the search bar of the config options .
- You will then see the entry: dom.ipc.plugins.enabled.npctrl.dll
- Now Change the value from true to false (just by double-clicking will change this).
- Now Restart Firefox
Thanks,
Maninder
Introduction to Validation in Silverlight 4 (Part 1)
Data validation is most important for data driven applications. This article talks about the basics of validation in Silverlight 4.
Following are some screen shots of how the sample application looks before and after the validation.
Before:
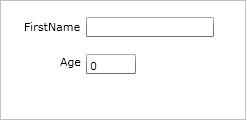
After:
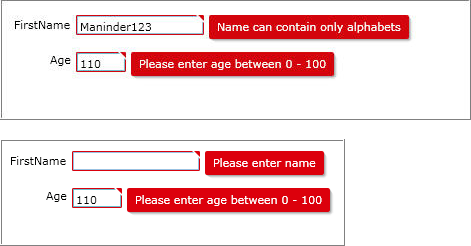
Following is an employee class which has two properties namely name and age. The properties of the employee class are validated whenever the user sets the properties. In this example, the following checks are made
For Name property : Name should not be empty and should contain only letters ("alphabets")
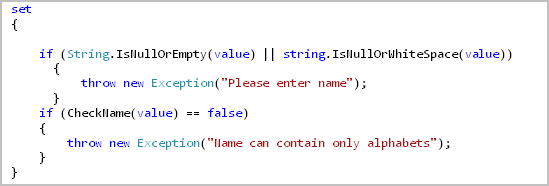
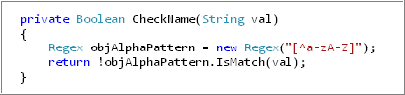
For Age property : Age should be between 0 - 100
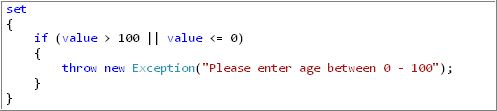
Following is the mainpage.xaml where you have to set the validation for the controls.
For textbox control accepting Name of employee

For textbox control accepting Age of employee

Subscribe to:
Posts (Atom)